12+ Things I Regret Not Knowing Earlier About Python Lists
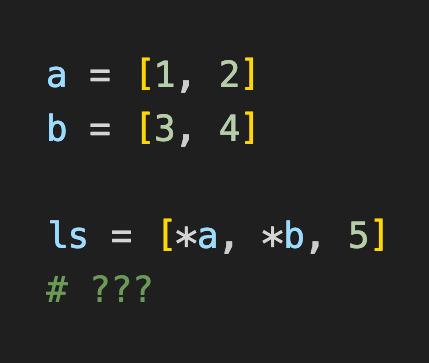
1) We can use * to combine lists
We can use * to combine lists together.
More specifically, when put in front of a list, the * causes the list to expand itself into its container (eg another list)
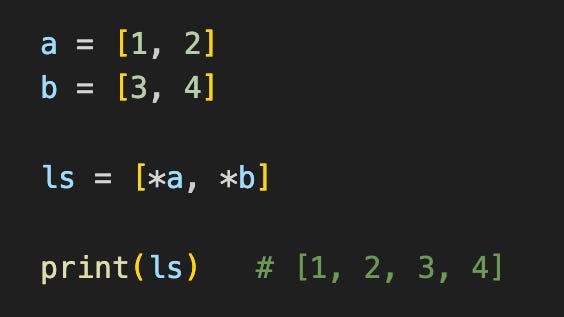
We can combine this with singular elements + choose which lists to expand and which not to.
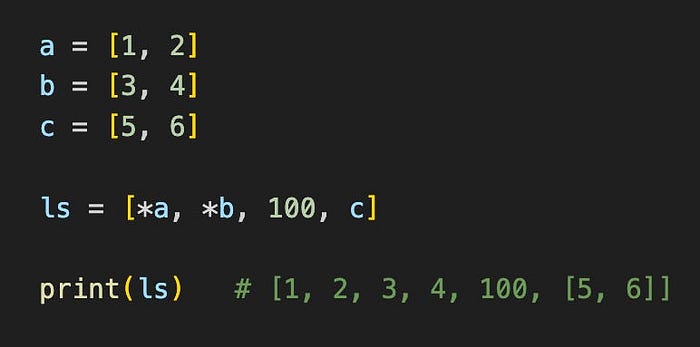
2) We can use * to unpack lists
Let’s say we have a list containing some dog info — the first element is its name, the second element is its age, and the rest are its favourite food.

We can use * to unpack a section of the list into a variable. For instance, we want the name and age individually, but the favourite food as a list.
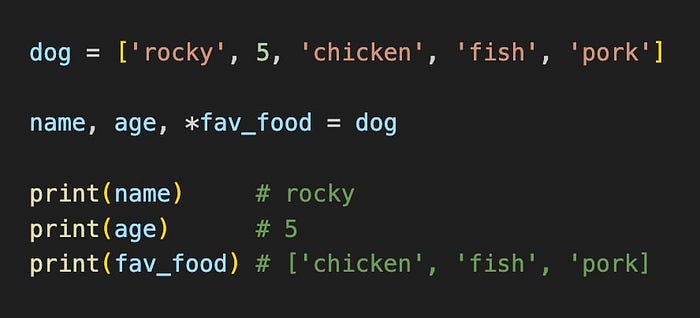
Here, rocky and 5 are assigned to name and age as they are the first 2 elements.
However, *fav_food is assigned to everything else and catches all unassigned elements as a list.
3) Using * unpack a list as function arguments
Let’s say we have a simple function that takes in (a, b, c) and prints them
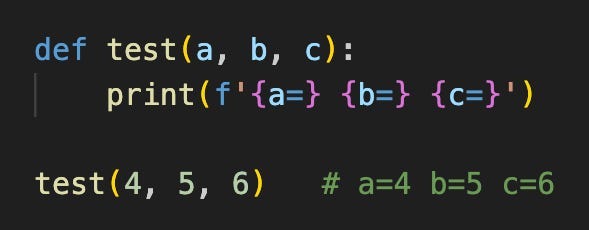
If we have a list containing 3 elements, we can pass it into this function using the * operator to unpack it. The * operator unpacks the list’s elements into its container.
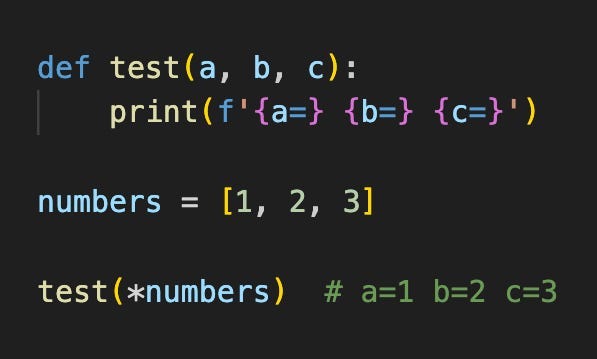
Here, given that numbers = [1, 2, 3], test(*numbers) is the same as test(1, 2, 3)
Note that in this case, test(a, b, c) takes in exactly 3 arguments, so our input list must contain exactly 3 arguments.
4) zip() to iterate through 2 lists concurrently
The built-in zip() function allows us to iterate through 1 or more lists concurrently:
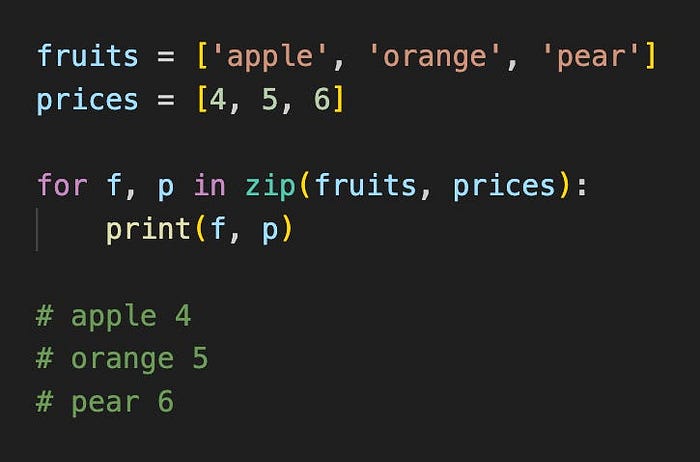
We can iterate through 3 or even more lists concurrently if we wish to:
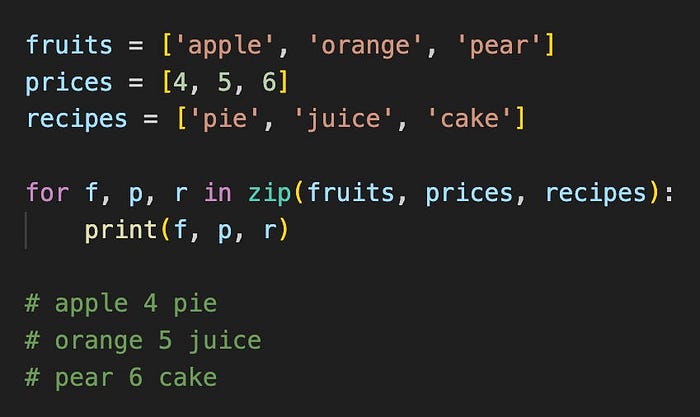
5) enumerate() to generate both index and value during iteration
If we wish to generate both the index and value of our list element during iteration, we can consider using the built-in enumerate() function.
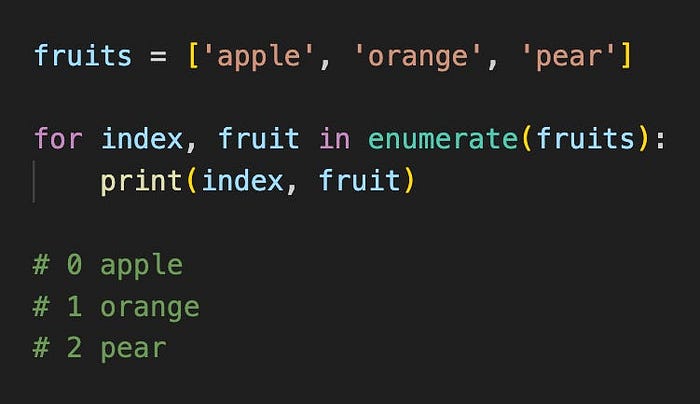
Quick Pause
I recently wrote a book 101 Things I Never Knew About Python detailing Python stuff I didn’t learn as early as I could have.
Check it out here: https://payhip.com/b/vywcf
6) .sort() vs sorted()
Both .sort() and sorted() sorts a list, but there is one key difference.
.sort() returns nothing and is done in place — meaning that the original list itself becomes sorted.
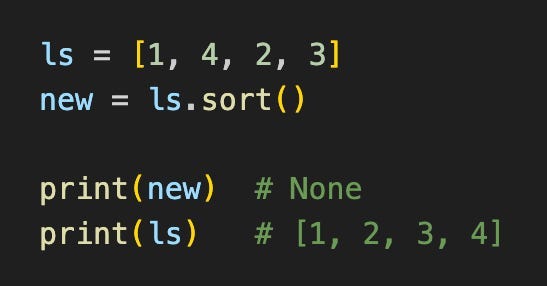
sorted() creates and returns a sorted copy of our list — meaning that the original list remains as it is and does not get changed. Which can be useful if we want to preserve the original order of things for some reason.
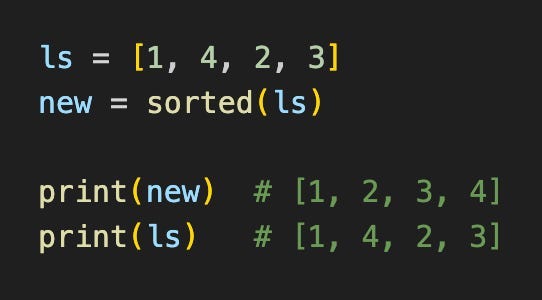
7) .sort() with custom condition
This works for both .sort() and sorted(), but let’s just use .sort() as our example. By default, .sort() sorts our numbers in ascending order .
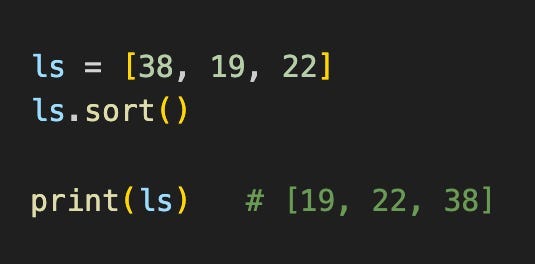
If we wish to sort by a custom condition, we can pass in our custom function into the key keyword argument. Let’s say we want to sort by the last digit to get [22, 38, 19]
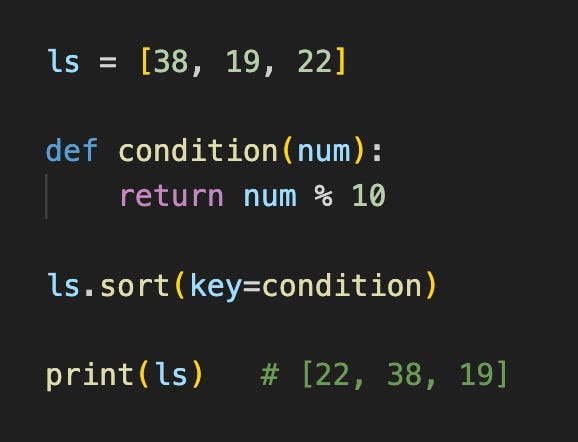
We can shorten this using an equivalent lambda function if we want to
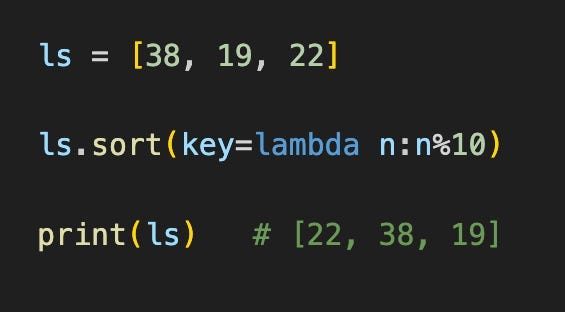
8) List comprehension
Let’s say we have a list of fruits, and we want to convert them into uppercase. This is one basic we which we might go about doing this:
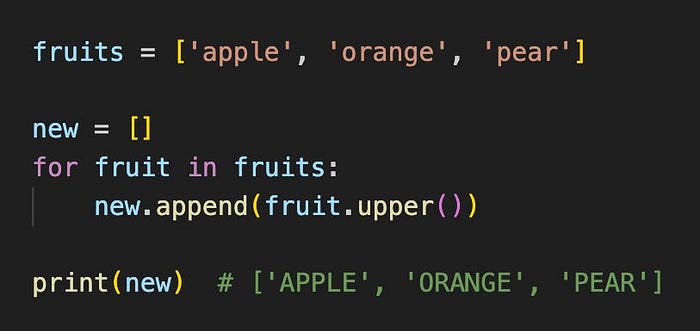
However, we can use list comprehension to do this more elegantly in one line of code. Here, the .upper() transformation is applied inside the list comprehension itself.
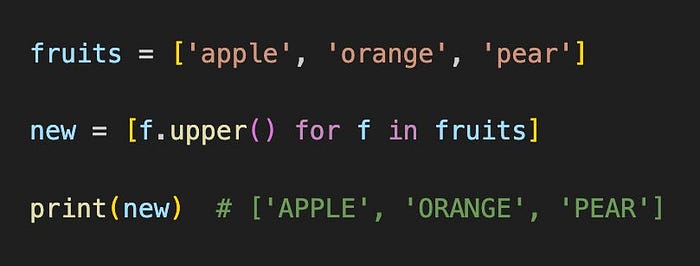
We can add a conditional statement to filter fruits that we wish to keep. For instance, let’s keep only fruits that have a length of 5 or more:
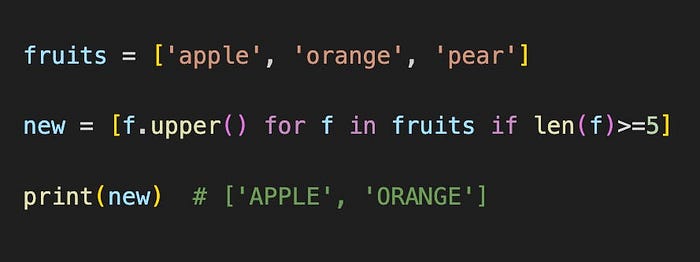
9) Tuples vs Lists
Tuples are essentially immutable lists. Which means that we cannot mutate our tuple (add/remove stuff) after we create our tuple.
We define a tuple using normal brackets instead of square brackets:
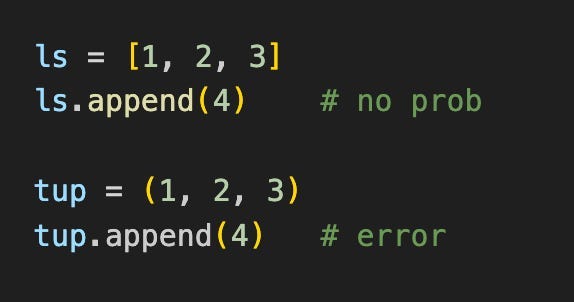
Disadvantages of using tuples over lists:
- because tuples are immutable, we cannot add new elements to our tuple
- because tuples are immutable, we cannot remove elements from our tuple
Advantages of using tuples over lists:
- tuples are immutable, which means they are hashable
- tuples are hashable, which means they can be used as dictionary keys (lists can’t)
- tuples are hashable, which means they can be added into a set (lists can’t)
10) The .insert(index, element) method
We are probably familiar with the .append() method of a list, which simply adds a new element to the back of a list.
But did you know that we also have the .insert() method, which puts a new element at any index we desire?
Inserting ‘AAA’ into index 0 — ‘AAA’ will be at index 0 in our list, and everything else will be pushed back by 1 index.
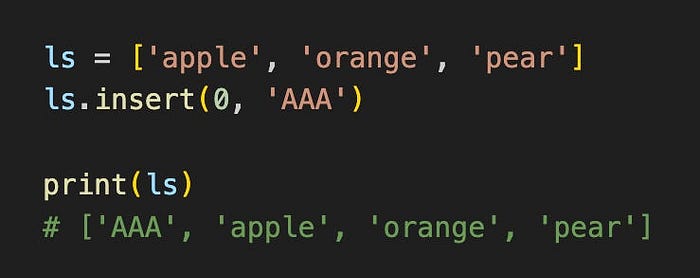
Inserting ‘AAA’ into index 1 — ‘AAA’ will be at index 1 in our list, and everything else will be pushed back by 1 index.
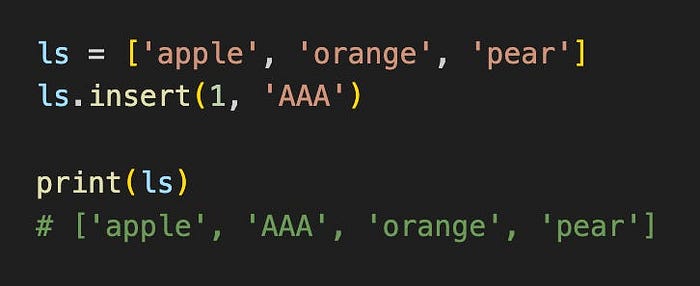
Note — all elements after our inserted index will be pushed back by 1 index, which means that this operation takes O(n) time, or has a linear time complexity. Which just means that this might be slow if we have many many elements in our list.
11) The .extend(other_list) method
We can use the .extend() method to add all elements in one list to the back of another.
Here in a.extend(b), we add everything from b into a. And b remains unchanged:
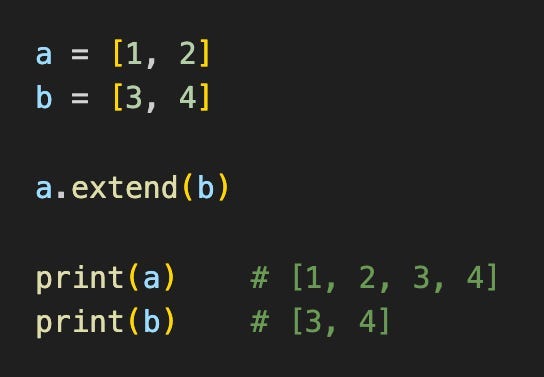
Technically, we can do a = a + b , but this method creates an entirely new list, then assigns it to a. Which makes this method less memory efficient.
12) list[start:end] = [1, 2, 3]
You probably know that we can replace elements in lists using list[index] = new_element.
But did you know that we can replace a range of elements this way?
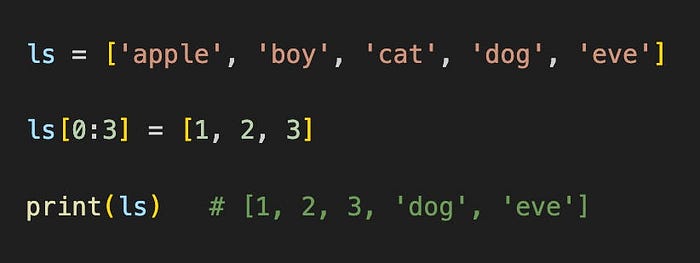
- here, ls[0:3] means [‘apple’, ‘boy’, ‘cat’]
- ls[0:3] = [1, 2, 3] means Python will replace [‘apple’, ‘boy’, ‘cat’] with [1, 2, 3]
Note — the lengths of the ranges do not need to match. We can replace a range of length 3 with only 1 element, just like below:
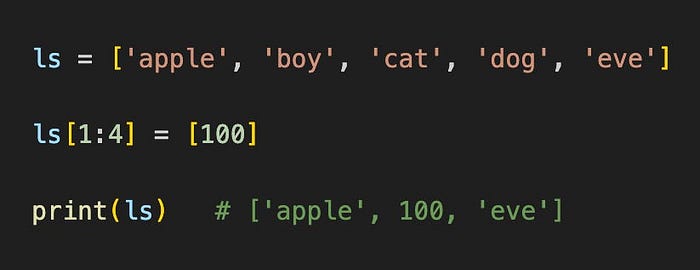
17 Things I Regret Not Knowing Earlier About Lists in Python
Do find the full post on substack and support me if you wish to!
If You Wish To Support Me As A Creator
- Join my Substack newsletter at https://zlliu.substack.com/ — I send weekly emails relating to Python
- Buy my book — 101 Things I Never Knew About Python at https://payhip.com/b/vywcf
- Clap 50 times for this story
- Leave a comment telling me your thoughts
- Highlight your favourite part of the story
Thank you! These tiny actions go a long way, and I really appreciate it!
YouTube: https://www.youtube.com/@zlliu246
LinkedIn: https://www.linkedin.com/in/zlliu/